ACT J anima
Animate elements in a webpage with simple commands.
Very easy to use and completely customizable. Can be used and adjusted just with HTML classes so you can easily use it in WordPress block editor. Just enque the script and use the AJ- classes on any element you want.
Features
- animate position, scale, rotation, fading, bluriness
- custom timing and easing function for each motion step
- combine motion steps for complicated animations
- animate children of selected element with delay between them
- animate on click, on load or on any custom event
Usage
using classes in HTML
You can animate elements, giving them classes that begin with AJ- and contain all the needed commands and values.
For example if an element has the class AJ-bottom-play, the element will be placed 100pixels (the default value) lower than its original position and on page load it will be animated back to it.
If the class is AJ-bottom:200-fade-play then the motion will be 200pixels and the fade command will hide the element on the beggining and fade it in.
If multiple AJ- classes exist, each one defines a motion step.
You can pass several commands and parameters in the AJ- class. The parameters must be seperated with a dash(-).
Every parameter can optionally have a value seperated with the name by a semicolon(:). If no value is given, then default value will be used.
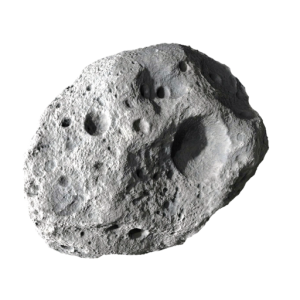
The asteroid has the following classes:
- AJ-scale:10-rot:360-fade-time:2-ease:elastic-play
- AJ-scale:150-rot:90-time:2-blur:10-ease:ease
- AJ-scale:100-rot:__360-time:2-blur:0-ease:elastic
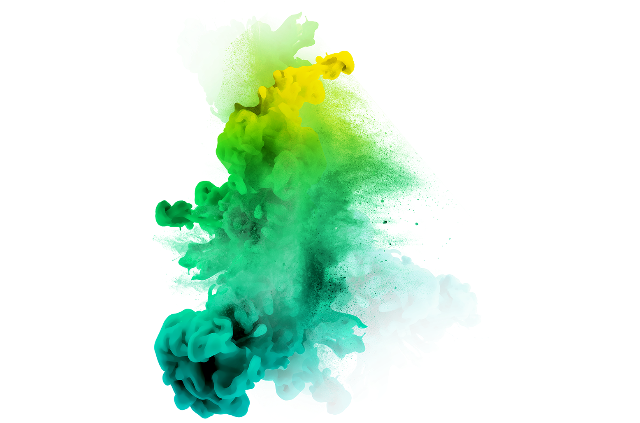
classes:
- AJ-x:600-play
- AJ-x:300
- AJ-x:__200
reload the page to replay the animation
<div class="AJ-bottom-fade"></div>
<div class="AJ-bottom:80-rot:30"></div>
<div class="AJ-scale:2-fade-time:2-ease:elastic-play"></div>
<div class="AJ-out-scale:2-left:100-fade-play:onClick"></div>
<div class="AJ-items-bottom-fade"></div>
<div class="AJ-items:0.3-bottom-fade-blur"></div>
<div class="AJ-bottom-fade-play AJ-left:200 AJ-top:80"></div>
<script type="text/javascript" src="ACT_J_anima.js"></script>
motion commands
- fade:0 [float]
- x:100
y:100
top:100
bottom:100
left:100
right:100 [integer] pixels
the distance of the animation. You can use either x/y and give positive or negative values, or you can use left/right/top/bottom with positive values to indicate the direction of the movement. Negative values should be passed in classes, using ‘__’ (double low dash) as the dash is used for seperating the commands - scale:200 [integer] size percentage
the scaling of the element - rot:45 [float] degrees
the rotation of the element in degrees - blur:10 [integer] pixels
the blur amount. The blur effect is “expensive” it terms of hardware and might be laggish on weaker machines. Use with care.
keyframe control parameters
- time:1 [floating] seconds
the animation duration in seconds - ease:ease [string] easeIn / easeOut / easeInOut / ease / linear / elastic / elasticOut
the animation easing function
animation control parameters
- items:0.1 [float] seconds
the animation will be applied to the target’s children.
If the argument is given in a class, the parameter defines the delay for every child’s animation.
WARNING: if set in a class, it must be the first parameter after aj- - delay:0.1 [number]
The delay between the animation of each child element (on items mode) - out
if set, the animation begins on the default element position and animates to the given values. By default the animation follows the opposite direction - play:onLoad [string] onLoad / onClick / customEvent
defines the event that triggers the animation playback. Combine with ACT reveal to play animation on element reveal.
Javascript
In javascript you can specify target elements and animation parameters in the given arguments.
The mode parameter defaults to ‘element‘. If you set it to ‘items‘, the animation will be applied to each of the target’s children with the given delay between them.
The animation array can contain one or more motion steps. The values for each motion step are defined like in the AJ- classes.
let myAnim = new ACTJanima({
target : '.my-element',
mode : 'items',
delay : 0.4,
play : 'onLoad',
animation : [
['bottom-rot:30-fade'],
['left:100-time:2'],
['top:60'],
],
})
myAnim.play()